An End-to-End Encrypted File Sharing System¶
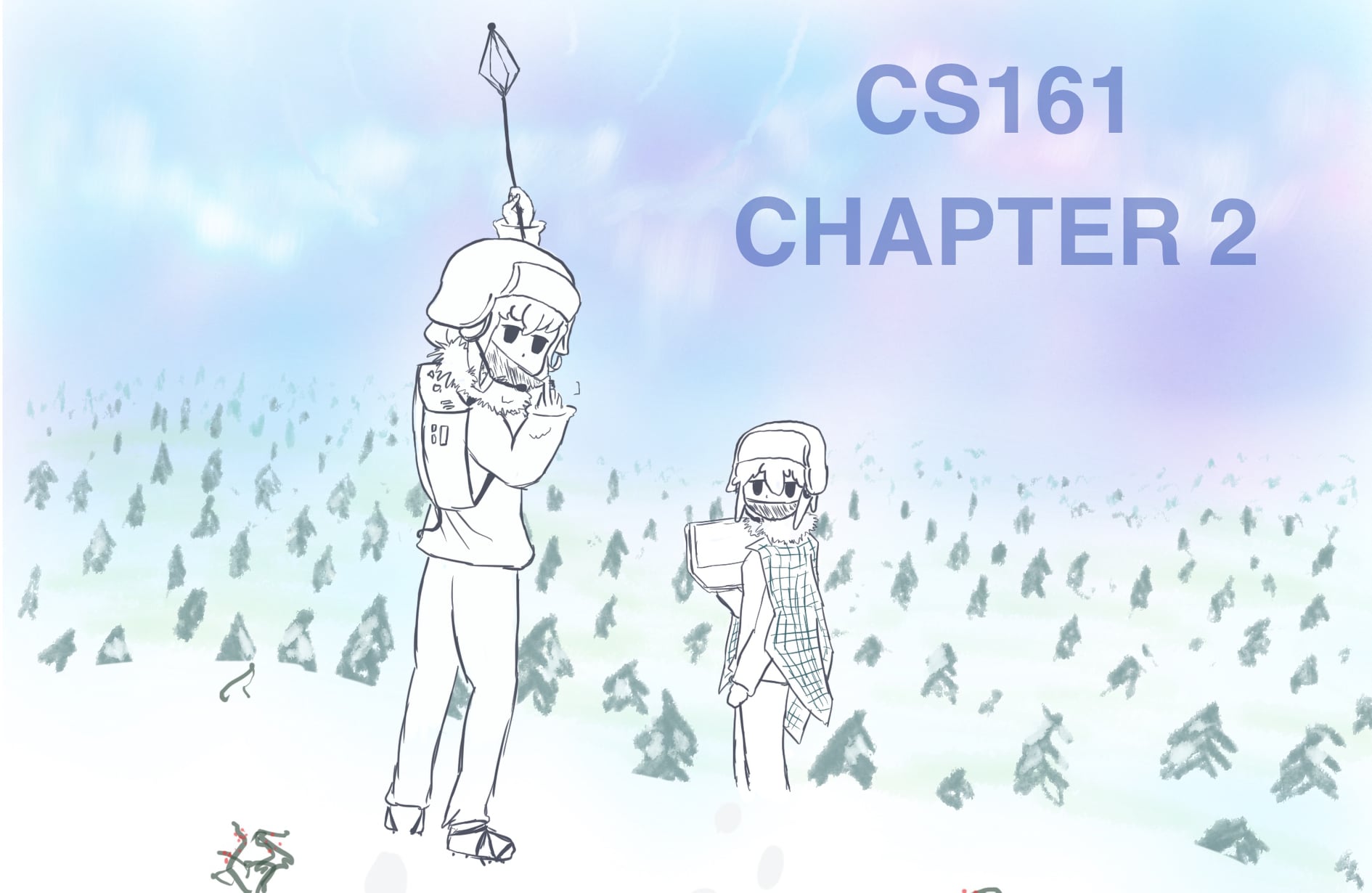
In this project, you will apply the cryptographic primitives introduced in class to design and implement the client application for a secure file sharing system. Imagine something similar to Dropbox, but secured with cryptography so that the server cannot view or tamper with your data.
The client will be written in Golang and will allow users to take the following actions:
Authenticate with a username and password;
Save files to the server;
Load saved files from the server;
Overwrite saved files on the server;
Append to saved files on the server;
Share saved files with other users; and
Revoke access to previously shared files.
We provide several resources to get you started.
We provide two servers that you can utilize in the design of your client application: the Keystore, and the Datastore.
We provide implementations of several cryptographic algorithms and a number of functions that you can use to interact with Keystore and Datastore. These utilities are defined in userlib, which is already imported into client.go.
The Project 2 - Starter Code defines 8 functions in client.go that you must implement (see Grading and Deliverables).
Using these resources and your knowledge of computer security, you will design a secure client application that satisfies all of the Design Requirements.
The best way to digest this project documentation is to read each section
sequentially using the Next
button at the bottom of each page.
As always, if you have questions about this documentation (or find errors), please make a post on Piazza!
Staff Advice¶
Design a solution before starting the implementation. Students consistently agree that design is harder than implementation across multiple iterations of this project. A faithful implementation of a faulty design will not earn you many points.
To approach the design process, read through the Design Requirements and the function definitions that you are required to implement in client.go. Think about how you can design your client to provide the required functionality. Here are some useful questions to get you started:
Where will you store data?
What data will be stored on which server?
What data structure will you use to store the data?
If you are stuck, try ignoring the file sharing functionality and instead focus on how to provide just the store/load file functionality. While you might need to later change your design to support secure sharing, this project is much easier to grasp when sharing is not involved.
Make sure your implementation does not panic on the basic functionality tests provided in client_test.go. An implementation that panics on those tests will get a zero in the code section.
Submit to the autograder once in a while. The autograder will warn you if your implementation panics in any of the hidden tests.
Contents¶
- 1. Grading and Deliverables
- 2. Threat Model
- 3. Design Requirements
- 4. Server APIs
- 5. Client Application API
- 5.1. InitUser: Create a user account.
- 5.2. GetUser: Log in using username and password
- 5.3. User.StoreFile: Store a new file
- 5.4. User.LoadFile: Load a previously stored file
- 5.5. User.AppendToFile: Efficiently append data to an existing file
- 5.6. User.CreateInvitation: Create a secure file share invitation
- 5.7. User.AcceptInvitation: Add a shared file to personal file namespace
- 5.8. User.RevokeAccess: Revoke file access
- 6. Some helpful examples
- 7. Cryptographic Functions
- 8. Getting Started Coding
- 9. Coding Tips
- 10. Changelog